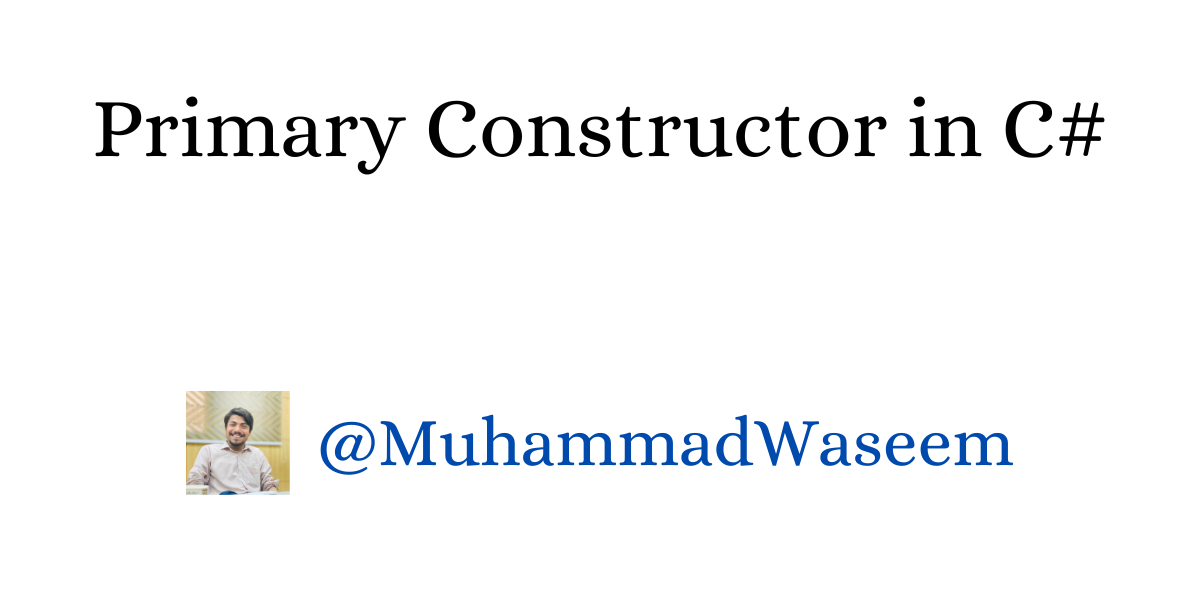
2 min read
Primary Constructor in C#
What is the primary constructor?
Primary constructors were introduced in .NET 8 and C# 12, a cleaner way of constructor initialization. We can use this syntax where we have a lot of constructor injections.
Understanding the Primary Constructor with Code
Letβs understand it with a simple example first before diving in depth. The older way of constructor injection:
public sealed class NewsletterService
{
private readonly INewsletterRepository _newsletterRepository;
public NewsletterService(INewsletterRepository newsletterRepository)
{
_newsletterRepository = newsletterRepository;
}
}
This is how we can achieve the same with primary constructors:
internal class NewsletterService(INewsletterRepository newsletterRepository)
{
public void Subscribe(string email)
{
newsletterRepository.Add(email);
}
}
Pro(s) :
The following are the benefits of using primary constructors :
-
It removes a lot of boilerplate code.
-
Simplifies the syntax.
-
Much more readability.
This article was originally published at https://mwaseemzakir.substack.com/ on .
Whenever you're ready, there are 3 ways I can help you:
- Subscribe to my youtube channel : For in-depth tutorials, coding tips, and industry insights.
- Promote yourself to 9,000+ subscribers : By sponsoring this newsletter
- Patreon community : Get access to all of my blogs and articles at one place