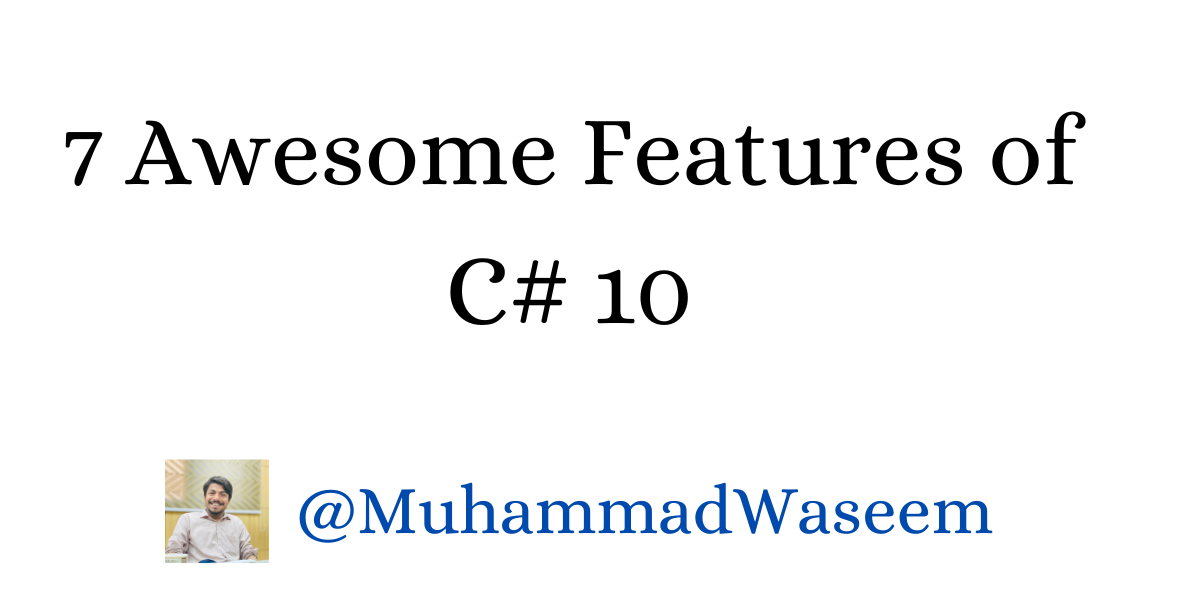
7 Awesome Features of C# 10
1. Lambda Expressions
Lambda expressions are used to create anonymous functions , I love to use them for simple getter/setters e.g. GetFullName , Get Address etc. But lambdas have limitations with var types which were entertained in C# 10.
Func<string>? newsletterName = () => "This is Waseem Newsletter";
var possible = () => "This was not possible before C# 10";
var firstName = null; // Not Possible
var lastName = string? () => null; // Possible with C# 10
2. Extending Properties
Extending property was introduced in C# 8.0 , it allow a property sub patterns to reference nested members, for instance
Engine Engine = new(2000, "V8");
Car bmw = new("AB1X", "BMW", Engine);
if (bmw is { Engine: { Capacity: > 2000 } })
{
Console.WriteLine("Awesome");
}
public record Engine(int Capacity, string? Type);
public record Car(string RegNo, string? Name, Engine? Engine);
Engine Engine = new(2000, "V8");
Car bmw = new("AB1X", "BMW", Engine);
if (bmw is { Engine.Capacity: > 2000 })
{
Console.WriteLine("Awesome");
}
public record Engine(int Capacity, string? Type);
public record Car(string RegNo, string? Name, Engine? Engine);
3. Global Using Statements
You would have seen your code loaded with a lot of using statements at the top , and some of statements are repeated in multiple files. Since C# 10 now we can get rid of them through following ways
- Either make a global class and define using statements in it
global using AutoMapper;
global using Microsoft.EntityFrameworkCore;
global using Microsoft. EntityFrameworkCore.Query;
global using Microsoft. Extensions.Dependency Injection;
global using System.ComponentModel;
global using System.Diagnostics.Contracts;
global using System.Reflection;
- Include your using statements in .csproject file
<Project Sdk="Microsoft.NET.Sdk">
<ItemGroup>
<Using Include="AutoMapper" />
<Using Include="Microsoft.EntityFrameworkCore"/>
</ItemGroup>
</Project>
4. File Scoped Namespaces
Now we can get rid of of namespaces and use file scoped namespaces.
namespace Learn; {
public class Program {
string name = "Muhammad Waseem"; int age = 98;
}
}
namespace Learn;
public class Program {
string name = "Muhammad Waseem"; int age = 98;
}
5. Structs Got The Freedom
Now structs can have parameter less constructor opposite to previous one like this.
public struct Student
{
public int Id { get; set; }
public string Name { get; set; }
// Constructor with parameters
public Student(int id, string name)
{
Id = id;
Name = name;
}
}
with can be used for structs as well now
var student = new Student(1, "Stefan");
var updated = new Student(student.RollNo, "Dr. Stefan");
public struct Student
{
public int RollNo { get; set; }
public string Name { get; set; }
public Student(int rollNo, string name)
{
RollNo = rollNo;
Name = name;
}
}
6. Seal .ToString() For Records
Before C# 10 we could not apply sealed keyword for ToString override against records but now we can do that.
public record BaseStudent(int RollNo, string Name)
{
// This method can be overridden by child
public override string ToString()
{
return $"RollNo = {RollNo}, Name = {Name}";
}
}
public record BaseStudent(int RollNo, string Name)
{
// Override ToString method in the base record class
public override string ToString()
{
return $"RollNo = {RollNo}, Name = {Name}";
}
}
Above mentioned feature was added in C# 10 for records.
7. Constant Interpolated Strings
If you don’t know let me remind you that we use $ with for our strings and then using { } we can define our variables that is string interpolation.
Previously we were unable to do this thing with constant strings , but now we can achieve it.
public static class Constants {
public const string FirstName = "Stefan";
public const int Age = 30;
public static string details = $"Name = {FirstName}, Age = {Age}";
}
Whenever you're ready, there are 3 ways I can help you:
- Subscribe to my youtube channel : For in-depth tutorials, coding tips, and industry insights.
- Promote yourself to 9,000+ subscribers : By sponsoring this newsletter
- Patreon community : Get access to all of my blogs and articles at one place