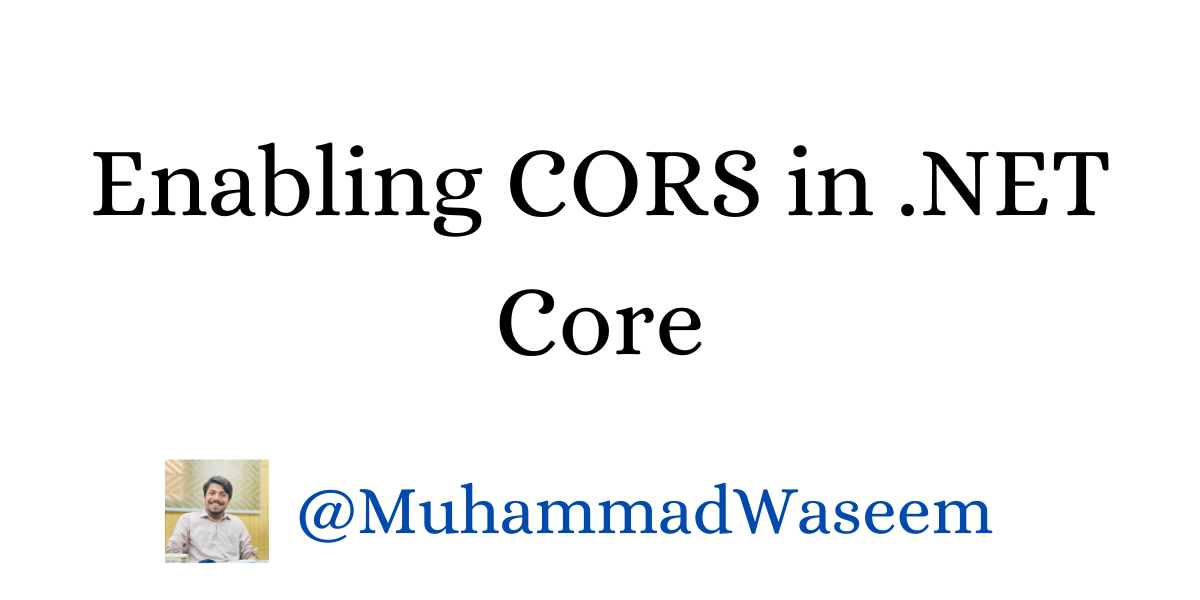
CORS and how to enable them in .NET Core ?
What is CORS
CORS stands for Cross Origin Resource sharing, so what exactly is cross origin, letβs understand with some dummy URLs.
These two URLs have the same origin
URL No 1 : https://www.facebook.com/GetFriends
URL No 2 : https://www.facebook.com/GetPosts
These URLs have different origins
URL No 1: https://www.facebook.com/GetFriends
URL No 2 :https://www.twitter.com/GetFollowers
Suppose we have an .NET Core Web API which is responsible for data and we have three different applications with different origins that use that API , so here comes the concept of CORS in action. We need to enable the CORS to
- Facilitate requests from different origins
- To get away from browsers same origin policy
- Browsers without CORS canβt do cross-origin requests.
Different available methods
In .NET 6 by using the combination of these methods we can enable CORS as per our need.
ππ₯π₯π¨π°ππ§π²ππ«π’π π’π§: This policy allows requests from any origin.
ππ’ππ‘ππ«π’π π’π§π¬: This policy allows requests from specific origins. You can specify one or more origins as arguments to this method.
ππ₯π₯π¨π°ππ§π²ππππππ«: This policy allows requests with any header.
ππ’ππ‘ππππππ«π¬: This policy allows requests with specific headers. You can specify one or more headers as arguments to this method.
ππ₯π₯π¨π°ππ§π²ππππ‘π¨π: This policy allows requests with any HTTP method (e.g., GET, POST, PUT, DELETE).
ππ’ππ‘ππππ‘π¨ππ¬: This policy allows requests with specific HTTP methods. You can specify one or more methods as arguments to this method.
How to enable them with and without restrictions
Following code demonstrates how to enable CORS and allow request from any origin with any header and any method in Program.cs
// Enable CORS, allow any Origin and get policy name from appSettings
var cors = builder.Configuration.GetSection("Cors");
builder.Services.AddCors(options =>
{
options.AddPolicy(name: cors.GetValue<string>("PolicyName"),
builder =>
{
builder.AllowAnyOrigin();
builder.AllowAnyHeader();
builder.AllowAnyMethod();
});
});
app.UseRouting();
app.UseAuthentication();
// Enable CORS
app.UseCors(cors.GetValue<string>("PolicyName"));
app.UseHttpsRedirection();
We can restrict those requests to few origins as well , following code displays how to allow only Get/Put requests from few origins.
Instead of getting policy name from appsetting we can pass direct string as well like
options.AddPolicy(name : β_AllowAnyOriginPolicyβ)
builder.Services.AddCors(options =>
{
options.AddPolicy(name: cors.GetValue<string>("PolicyName"), builder =>
{
builder.WithOrigins("https://hakuna.com",
"https://hakuna.net",
"https://hakuna.edu");
builder.AllowAnyHeader();
builder.WithMethods("Get", "Put");
});
});
Important things to keep in mind about CORS
βοΈCORS is not a security feature. CORS is a W3C standard that allows a server to relax the same-origin policy.
βοΈAn API isnβt safer by allowing CORS.
Whenever you're ready, there are 3 ways I can help you:
- Subscribe to my youtube channel : For in-depth tutorials, coding tips, and industry insights.
- Promote yourself to 9,000+ subscribers : By sponsoring this newsletter
- Patreon community : Get access to all of my blogs and articles at one place