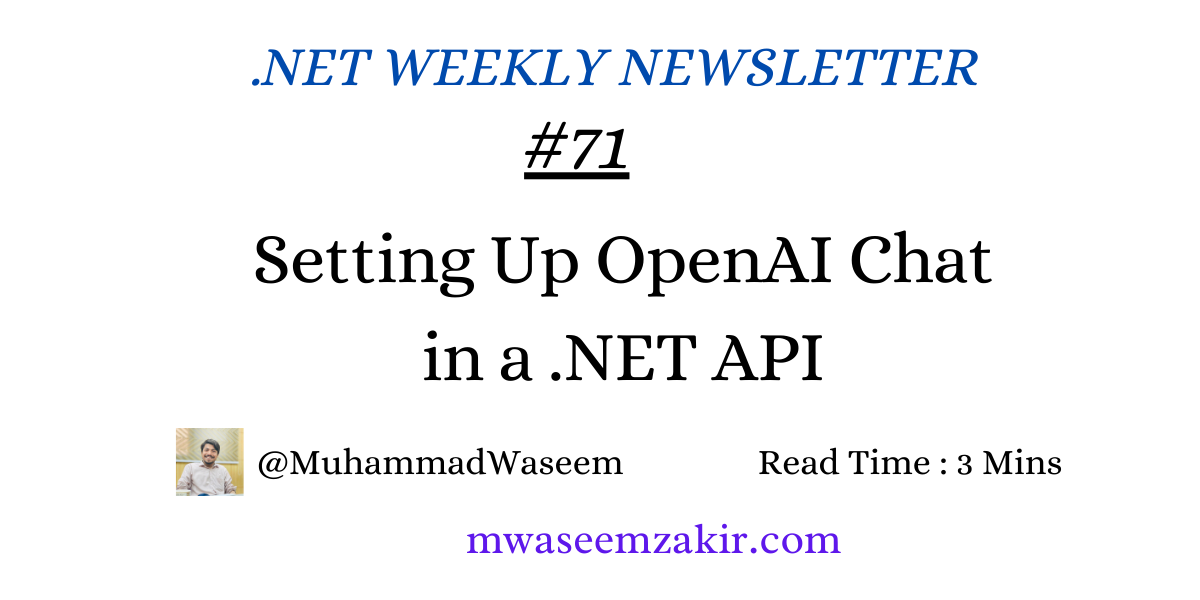
Setting Up OpenAI Chat in a .NET API
AI is everywhere!
Let’s walk through three simple steps to add it to your Web App or Web API :
Get your API Key
Retrieve your API key from the OpenAI website. Make sure you have already created the account.
Install the Required NuGet Package
Add the OpenAI NuGet package to your .NET API project :
dotnet add package OpenAI
Configure Your Code
Set up your code to initialize and use the OpenAI API, this is the sample code
public async Task<string> Chat(string query)
{
ChatClient client = new(model: "gpt-4o", apiKey: API_KEY);
ChatCompletion completion = await client.CompleteChatAsync(query);
string response = completion.Content[0].Text;
return response;
}
This code’s purpose is to demonstrate how we can connect the Open AI, we will see in the next sections how we can improve this code.
Available Methods and Properties
ChatClient comes from the OpenAI.Chat and the ChatClient constructor can take:
-
Model and ApiKey
-
Model and ApiKeyCredential
-
Model, ApiKeyCredential and OpenAIClientOptions
It has the following methods:
Task<ClientResult<ChatCompletion>>CompleteChatAsync(
IEnumerable<ChatMessage> messages,
ChatCompletionOptions options = null,
CancellationToken cancellationToken = default);
ClientResult<ChatCompletion> CompleteChat(
IEnumerable<ChatMessage> messages,
ChatCompletionOptions options = null,
CancellationToken cancellationToken = default);
Task<ClientResult<ChatCompletion>> CompleteChatAsync(
params ChatMessage[] messages);
ClientResult<ChatCompletion> CompleteChat(params ChatMessage[] messages);
Each method has its Async version as well we can use the one according to our need.
There is an additional asynchronous method called CompleteChatStreaming. Unlike CompleteChatAsync, this method streams the completion back token by token as it is generated.
ChatCompletion contains Id, Model, SystemFingerprint, Role, Content , ToolCalls and Usage details.
Listing every detail isn’t feasible, but our response is found within the Content object. This object includes Text, Refusal, and additional properties related to images if the response contains one.
Best Practices
Let’s improve this code :
public async Task<string> Chat(string query)
{
ChatClient client = new(model: "gpt-4o", apiKey: API_KEY);
ChatCompletion completion = await client.CompleteChatAsync(query);
string response = completion.Content[0].Text;
return response;
}
-
Create an Enum to define the available models rather than passing the model as a string.
-
Retrieve the API_Key from app settings or a secure vault.
-
Implement a dedicated service for OpenAI features to ensure reusability.
-
Provide separate methods for asynchronous and synchronous calls.
-
Perform NULL checks to validate the query.
-
Use try-catch blocks to handle exceptions.
-
Refer to OpenAI’s documentation to display detailed error messages for each error code :
Common Errors in Package Configuration
While setting up the library you can get this error :
The model gpt-4o does not exist or you do not have access to it.
To resolve this you must have a five USS balance in your Open API account.
Whenever you're ready, there are 3 ways I can help you:
- Subscribe to my youtube channel : For in-depth tutorials, coding tips, and industry insights.
- Promote yourself to 9,000+ subscribers : By sponsoring this newsletter
- Patreon community : Get access to all of my blogs and articles at one place