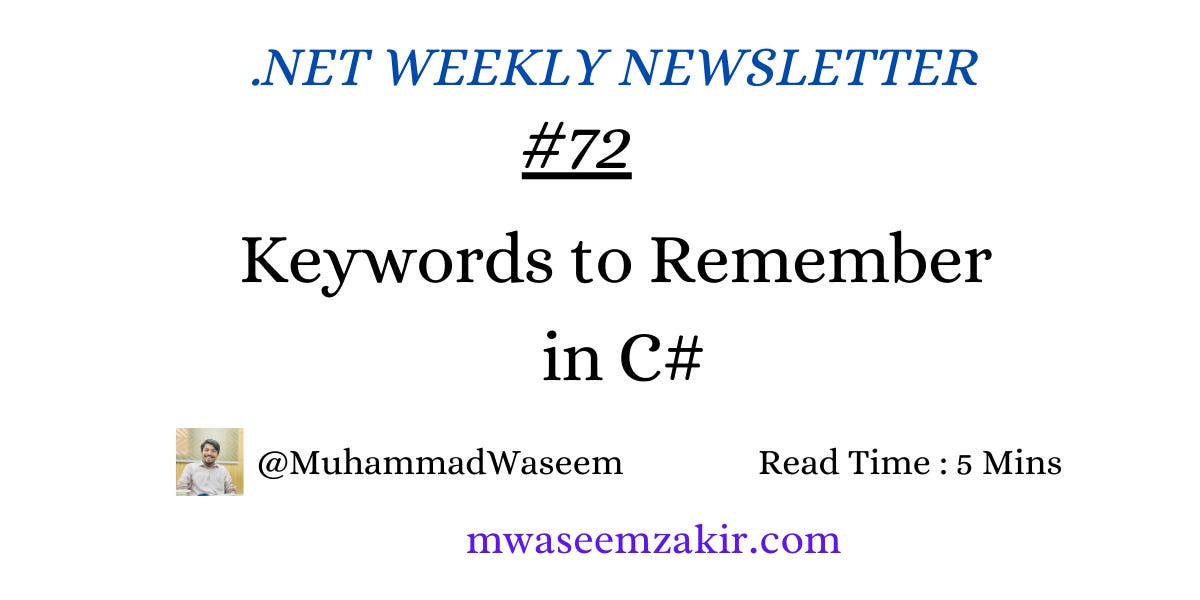
Keywords to Remember in C#
1/ Static
The static keyword is used to declare members that belong to the type itself rather than to any specific instance. This means you can access static members without creating an instance of the class. Static members are shared across all instances of the class :
public class Program
{
public static void Main(string[] args)
{
double squareOfFour = MathUtilities.Square(4);
}
}
public class MathUtilities
{
public static double Square(double number)
{
return number * number;
}
}
2/ Constant
The const keyword is used to declare a constant field or local. Constants are immutable values that are known at compile time and do not change for the life of the program. They must be initialized at the time of declaration.
public class Circle
{
public const double Pi = 3.14159;
public double CalculateCircumference(double radius)
{
return 2 * Pi * radius;
}
}
3/ Readonly
The readonly keyword is used to declare a field that can only be assigned during its declaration or in the constructor of the same class. This is useful for fields that should not change after the object is constructed.
public class Configuration
{
public readonly string _configName;
public Configuration(string name)
{
_configName = name;
}
}
4/ Async and Await
The async and await keywords are used to write asynchronous code. async is used to declare a method as asynchronous, and await is used to pause the execution of the method until the awaited task completes. This allows for non-blocking operations, improving the responsiveness of applications.
public async Task<string> FetchDataAsync()
{
await Task.Delay(1000);
return "Data fetched";
}
5/ Yield
The yield keyword is used in iterator methods to provide a value to the enumerator object and to signal the position in the code to resume execution when the next element is requested. It simplifies the implementation of iterators.
public IEnumerable<int> GetNumbers()
{
for (int i = 0; i < 5; i++)
{
yield return i;
}
}
6/ Ref
The ref keyword is used to pass arguments by reference, allowing the method to modify the original variable:
public class Program
{
public static void Main(string[] args)
{
int number = 5;
DoubleValue(ref number);
Console.WriteLine($"{number}");
}
public static void DoubleValue(ref int number)
{
number *= 2;
}
}
---
10
7/ Out
Similar to ref, the out keyword is used to pass arguments by reference, but it requires the method to assign a value to the parameter.
public class Program
{
public static void Main(string[] args)
{
if (TryParseNumber("123", out int result))
{
Console.WriteLine($"Parsed number: {result}");
}
else
{
Console.WriteLine("Failed to parse number.");
}
}
public static bool TryParseNumber(string input, out int result)
{
return int.TryParse(input, out result);
}
}
---
Parsed number: 123
8/ Params
The params keyword allows you to pass a variable number of arguments to a method:
public class Program
{
public static void Main((string[] args)
{
int sum = Sum(1, 2, 3, 4, 5);
Console.WriteLine($"Sum: {sum}");
}
public static int Sum(params int[] numbers)
{
int sum = 0;
foreach (int number in numbers)
{
sum += number;
}
return sum;
}
}
---
Sum: 15
9/ Is and As
The is keyword checks if an object is of a specific type.
The as keyword is used to perform safe type conversions, returning null if the conversion fails:
public void CheckType(object obj)
{
if (obj is string)
{
Console.WriteLine("It's a string!");
}
string str = obj as string;
if (str != null)
{
Console.WriteLine("Conversion successful!");
}
}
10/ Lock
The lock keyword is used to ensure that a block of code runs exclusively in a multi-threaded environment.
private readonly object _lock = new object();
public void ThreadSafeMethod()
{
lock (_lock)
{
// Critical section
}
}
11/ Base
base refers to the base class constructor or members:
public class BaseClass
{
public virtual void Display()
{
Console.WriteLine("Base class display");
}
}
public class DerivedClass : BaseClass
{
public override void Display()
{
base.Display();
Console.WriteLine("Derived class display");
}
}
12/ This
this Refers to the current instance of the class.
public class Person
{
private string name;
public Person(string name)
{
this.name = name;
}
}
13/ New
new keyword hides a member inherited from a base class or creates an object :
public class BaseClass
{
public void Display()
{
Console.WriteLine("Base class display");
}
}
public class DerivedClass : BaseClass
{
public new void Display()
{
Console.WriteLine("Derived class display");
}
}
14/ Abstract
abstract defines a class or method that must be implemented by derived classes.
public abstract class Shape
{
public abstract double Area();
}
public class Circle : Shape
{
private double radius;
public Circle(double radius)
{
this.radius = radius;
}
public override double Area()
{
return Math.PI * radius * radius;
}
}
15/ Sealed
sealed keyword prevents a class from being inherited.
public sealed class FinalClass
{
public void Display()
{
Console.WriteLine("This class cannot be inherited");
}
}
16/ Override
override keyword overrides a virtual method in a base class :
public class BaseClass
{
public virtual void Display()
{
Console.WriteLine("Base class display");
}
}
public class DerivedClass : BaseClass
{
public override void Display()
{
Console.WriteLine("Derived class display");
}
}
17/ Partial
partial keyword splits a class, struct, or method definition across multiple files.
// File1.cs
public partial class PartialClass
{
public void Method1()
{
Console.WriteLine("Method1");
}
}
// File2.cs
public partial class PartialClass
{
public void Method2()
{
Console.WriteLine("Method2");
}
}
Whenever you're ready, there are 3 ways I can help you:
- Subscribe to my youtube channel : For in-depth tutorials, coding tips, and industry insights.
- Promote yourself to 9,000+ subscribers : By sponsoring this newsletter
- Patreon community : Get access to all of my blogs and articles at one place